KYB Elements
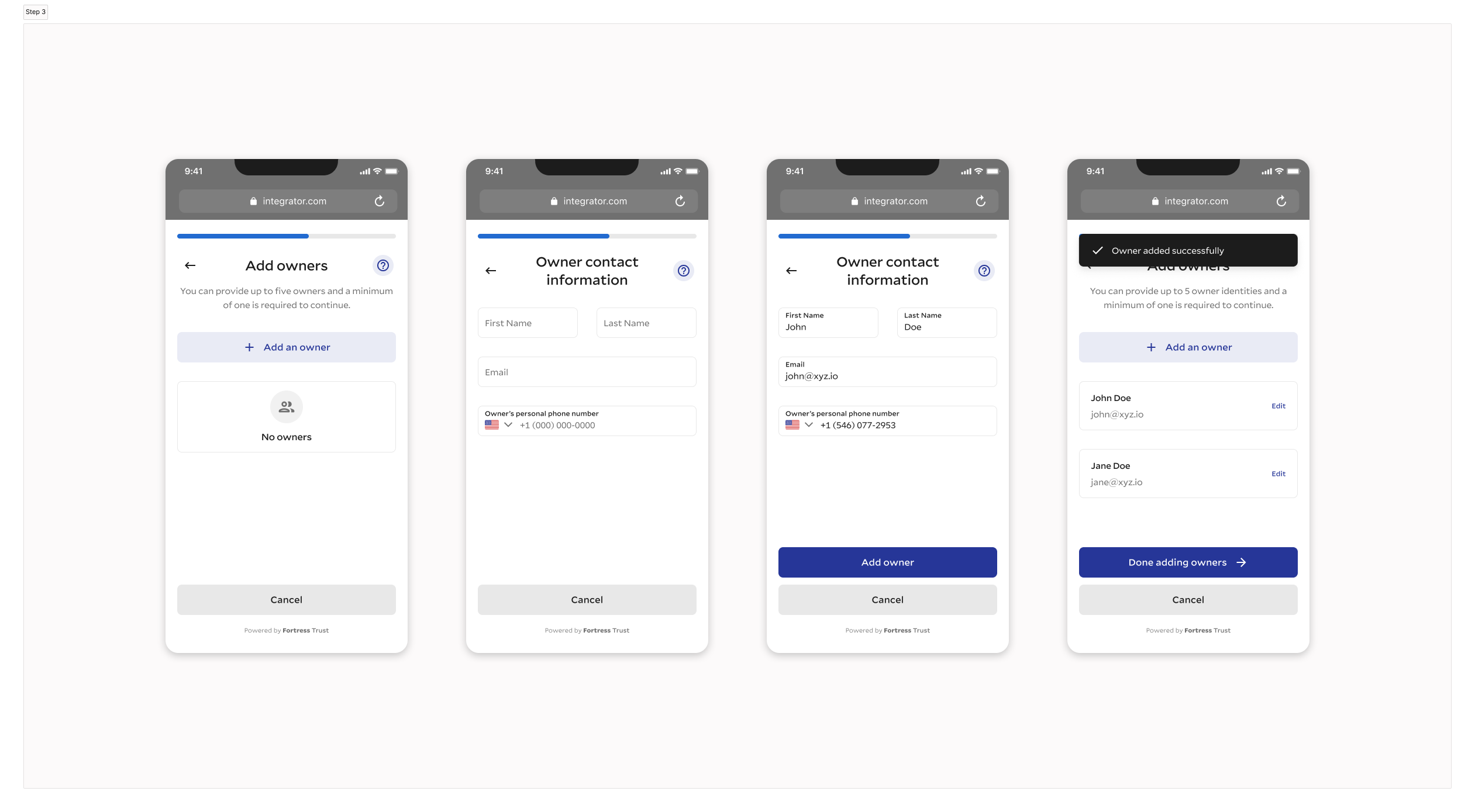
Default Identity Container
To utilize the KYB element, you must create a Default Identity Container for your Organization. All Entities created using the KYB element will be created under this Identity Container.
Note: This default container only ever needs to be created once per organization.
POST /api/trust/v1/identity-elements/default-business-identity-containers
//Sample Request
{
"firstName": "John",
"lastName": "Doe",
"email": "[email protected]",
"phone": "+15553332222"
}
KYB Element Specific Parameters
const EJS = window.FortressElementsJS;
const ElementKYB = EJS.createElementClient({
elementName: EJS.ElementNames.KYB,
onMessage: (message) => {
if (message.type === EJS .ElementEvenTypes.ELEMENT_STARTED) {
// handle action element started
}
},
theme: ThemeConfig, // link on ThemeConfig section
requiredKYBLevel: 'L1' | 'L2', // not required (L1 - default)
contactUsUrl: "https://someurl.com"
contactUsDescription: "If you need help, please contact us."
contactUsLabel: "Need help" // not required ("Contact us" - default)
});
Element_Updated Specific Messages
type ElementResult = {
status: initial | success| review | failed;
business?: {
identityId: '9a443475-5159-4f51-bf21-002e6609091e'
identityLevel: 'L0' | 'L1' | 'L2';
}
};
Complete React, TypeScript Code Sample
import React, { useEffect, useState, useMemo } from 'react';
import {ElementResult, WindowWithFortressElementsJS, ElementMessage } from './FortressElements'
const EJS = (window as WindowWithFortressElementsJS).FortressElementsJS;
function generateElementSessionJWT(identityId) {
if (!identityId) {
return axios.get('/api/trust/v1/identity-elements/kyb-jwt')
}
return axios.get(
`/api/trust/v1/identity-elements/{identityId}/jwt?element="kyb"`,
);
}
function Main() {
const ElementKYB = useMemo(() => EJS.createElementClient({
elementName: EJS.ElementNames.KYB,
onMessage: (message) => {
if (message.type === EJS.ElementEvenTypes.ELEMENT_STARTED) {
// handle action element started
}
},
theme: ThemeConfig // link on ThemeConfig section
}), []);
useEffect(() => {
ElementKYB.done(({ status, business }) => {
if (status !== EJS.ElementResultStatus.Success) {
// do something with business.identityId
// do something with business.identityLevel
}
console.log(`Element result status: ${status}`);
ElementKYB.destroy();
});
return () => {
ElementKYB.destroy();
};
}, [ElementKYB]);
const handleClick = async () => {
const identityId = '9a443475-5159-4f51-bf21-002e6609091e';
const { data: { jwt } } = await generateElementSessionJWT(identityId);
ElementKYB.run(jwt)
}
return (
<button
type="button"
onClick={handleClick}
>
Open Element Instance
</button>
);
}
export default Main;
Updated 5 months ago