Elements (Wire Widget)
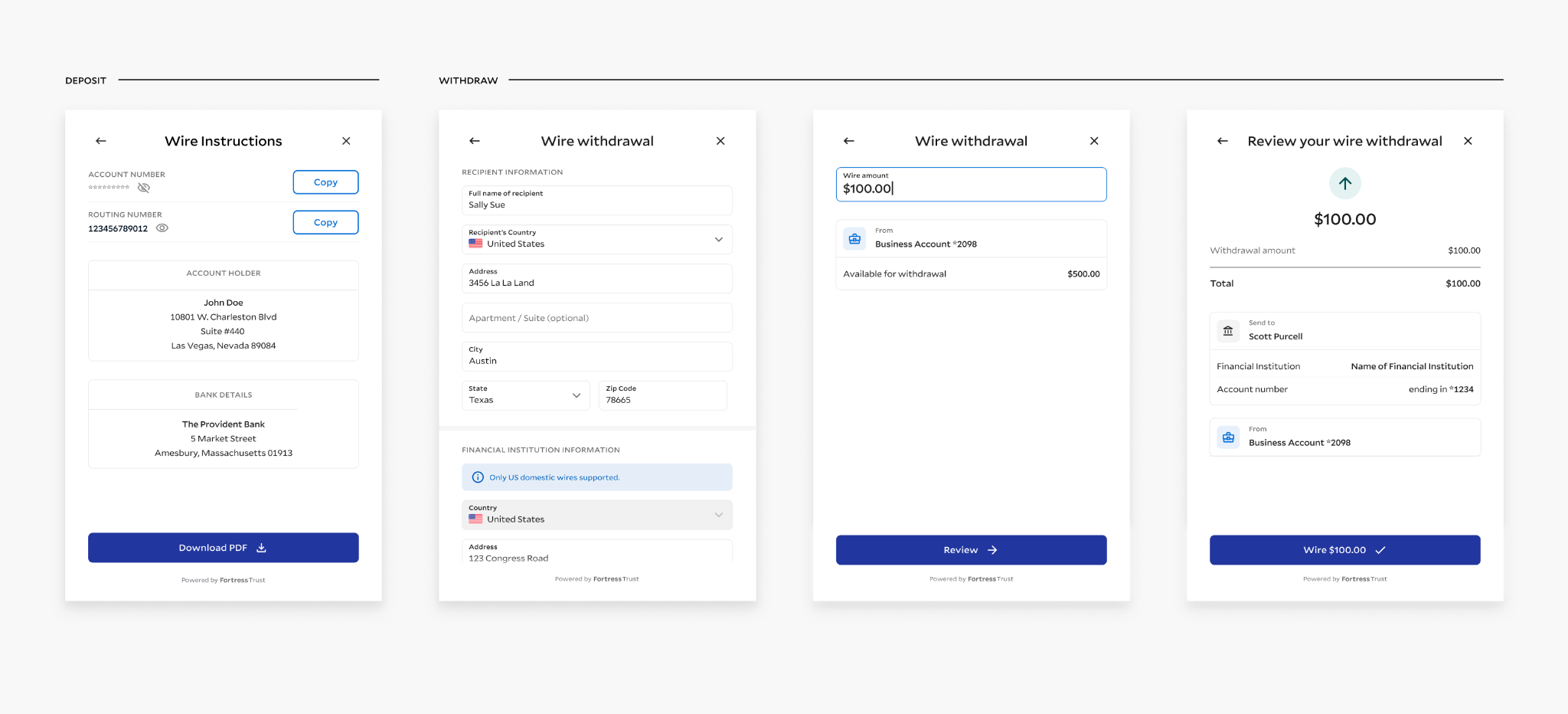
Need to deposit or send a wire fast? our Wire Elements lets end users generate deposit instructions for their custodial accounts, or withdraw money to a bank they connect.
Elements JS Library and Instance
Add a script tag with the corresponding URL for the Fortress file storage https://elements.fortressapi.com/libs. If there is a need to use some Environment specific build add the corresponding subdomain name: sandbox
, stage
e.g. elements.sandbox.fortressapi.com.
Do NOT put any async or defer attributes in tag. It may lead to unexpected issues with element.
<html>
<head>
<script src="https://elements.sandbox.fortressapi.com/libs/fortress-elements-js.min.js"></script>
</head>
</html>
Initialize The Elements Widget
After downloading the library, FortressElementsJS
will be accessible in the window global scope. It contains the methods responsible for creating the ElementClient
and manipulating/interacting with Fortress Public API. createElementClient
is returning the client instance.
const EJS = window.FortressElementsJS;
// `elementClient` keeps the reference to client
const elementClient = EJS.createElementClient({
elementName: EJS.ElementNames.TRANSFER,
onMessage: (message: ElementMessage) => {},
theme: { primaryColor: '#D70E0E', secondaryColor: '#CCC' },
uiLabels: {
statusScreenButton: "abc.."
}
paymentMethodTypes: 'Wire'
transferDirection: 'Deposit+Withdrawal' | 'Deposit' | 'Withdrawal'
});
Elements Authorization
Each instance of the elements widget will need a generated JWT token in order to run. Each new end user you onboard will need a JWT generated for them once the TRANSFER flow starts. The JWT expire time is 1 hour from generation. In order to generate the JWT, you'll have had to already created an L0
Identity.
Note: If the JWT expires during the TRANSFER workflow, the form will error out on submission and a new instance will need to be spun up for the end user to run through again.
GET /api/trust/v1/identity-elements/{identityId}/jwt?element="transfer"
//Sample Response
{
"jwt": "ey..."
}
Receive Transfer flow result
type ElementResult = {
status: initial | success| review | failed;
tradePayment: {
wireDomesticWithdrawalPaymentId: string | undefined;
wireInternationalWithdrawalPaymentId: string | undefined;
}
};
Updated 5 months ago